If a programmer has worked with languages such as C # or Java, he knows that in order to declare a variable, it is necessary to set the statistical type of data that will be stored in the specified variable from the very beginning. In the case when you need to change the value of the specified variable to another type, the system will throw an error. In contrast to them, JavaScript has a dynamic data type and there is no need to specify what type this variable will store, since in the process of executing the context, JS will try to solve the problem in accordance with the given values.
JavaScript data basics
JS data is divided into two groups: primitive and objects. The first includes a text line (String), logical data - true or false (Logical) and numbers (Number). There are two more special primitive types: Null and Undefined. They demonstrate interconnected, unordered JavaScript data types that have a name and have properties.
The object is specially created by the programmer, among them there are some special ones: global, prototype, arrays, functions, class, JS predefined classes, RegExp class for managing regular expressions and finding patterns in text, an error class for storing information related to errors. The first programming languages did not use objects, but only variables.
Variables are the computer’s memory space to which developers assign content mainly with a numeric value, character type, or character string — an alphanumeric value consisting only of text mixed with numbers. As an example of a JavaScript data type, you can define the variable “a”, which contains 32, and write it as:
a = 32.
Then you can change the value of “a” and do:
a = 78.
Or make “a” equivalent to the value of another variable “b”, for example:
a = b
Variables are elementary objects: a number, a symbol, a true or false value, while objects are complex, which can be formed by a large amount of information. But both forms occupy the same memory space, which can be more or less large.
Primitive types
Unlike objects, JavaScript primitive data types are not referenced, that is, when they make an assignment of a value from another variable.
In JS, there are such primitive types:
- brain teaser;
- numerical
- lowercase
- zero;
- undefined.
Booleans represent one of: true or false.
- var boo1 = true;
- var boo2 = false;
There is only one type of number in JS; it can be written with or without a decimal point. The number also happens:
- + Infinity;
- -Infinity;
- NaN (not a number).
var num1 = 32;
var num2 = + Infinity;
Lines are used to save text. They must be inside double or single quotes. In JS, strings are immutable.
var str1 = 'hello it's me';
var str2 = "hello it's me";
A variable that does not matter is not defined.
var test Var;
console.log (testVar); // undefined
Boolean is a data type that returns one of two things: true / false. The Boolean JavaScript data type is named after the mathematician George Boole, so it is always capitalized. You can demonstrate the principle of operation of this type on an example:
- Open the JS console and enter each of the following statements by pressing “Enter” after each action to see the result.
- Note that a single line comment is used after each statement to explain what it means.
- 1 <10 // 1 less than 10?
- 100> 2000 // 100 more than 2000?
- 2 === 2 // 2 exactly equal to 2?
- false === false //
The Boolean function returns the boolean value of a variable. It can also be used to find the logical result of a condition and expression; this allows JavaScript to use a functional data type.
Immutable characters
Symbols are a new primitive type in JS. They are created using the function:
const mySymbol = Symbol ('mySymbol').
Each time a programmer calls this function, a new and unique character is created. They can be used as constants to represent concepts such as colors. Symbols are mainly used as property keys and never conflict with any other key - a character or string. For example, you can make an object iterative, used through the for-of loop and other language mechanisms, using the symbol stored in Symbol.iterator as a key.
In line A, the character is used as the method key. This unique marker makes the object repeatable and allows the use of a for-of loop. It is not possible to cast characters to strings because there is coercion or implicit conversion of JavaScript data types. The prohibition of coercion prevents some errors, but also complicates the work with characters.
A new type of primitive is tokens that serve as unique identifiers. The programmer creates characters using the factory function "Symbol ()", which is slightly similar to "String" - returning strings when called by a function:
const symbol1 = Symbol ();
Symbol () has an optional string parameter that allows the newly created symbol to be described. This description is used when a character is converted to a string through “toString ()” or “String ()”.
Arithmetic operators
JS, like all programming languages, has a built-in ability of mathematical actions, like a calculator. They execute them on numbers or variables that are represented as numbers.
The addition operator, represented by a plus sign (+), adds two values and returns the sum.
var x = 2 + 2; // x returns 4
The subtraction operator, represented by a minus sign (-), subtracts two values and returns the difference.
var x = 10 - 7; // x returns 3
The multiplication operator, represented by an asterisk (*), multiplies two values and returns the product.
var x = 4 * 5; // x returns 20
The division operator, represented by a slash (/), separates the two values and returns the quotient.
var x = 20/2; // x returns 10
Less familiar is the module operator, which returns the remainder after division and is represented by a percent sign (%).
var x = 10% 3; // returns 1
This means that “3” goes into “10” three times, with a “1” remainder.
The increment performs an action in which the number will be increased by one using the increment operator, represented by the double plus sign (++).
var x = 10; x ++; // x returns 11
This happens after an appointment. You can also write what happens before the appointment. For comparison:
++ x;
var x = 10;
var y = x ++;
// y is 10, x is 11;
as well as:
var x = 10;
var y = ++ x;
// y is 11, x is 11.
Decrement - the number will be reduced by one using the decrement operator, represented by a double minus sign (-).
var x = 10; x--;
// x returns 9
As above, this can also be written: - x;
Varieties of JS operators:
- assignment operator;
- arithmetic increment and decrement operators;
- equality operators;
- relational operators;
- logical operators.
True or false functions
Comparison operators will evaluate the equality or difference of two values and return “true” or “false”. They are commonly used in logical statements. Two equal signs (==) mean equal in JS. It is easy for novice programmers to confuse between single, double and triple equal signs, you need to remember that one equal sign applies a value to a variable and never evaluates equality.
var x = 8;
var y = 8;
x == y; // true
This is a free equality type and will return true even if a string is used instead of a number.
var x = 8;
var y = "8";
x == y; // true
Three equal signs (===) mean strict equality in JS.
var x = 8;
var y = 8;
x === y; // true
This is a more frequently used and more accurate form of determining equality than the regular “equal (==)” function, since returning requires the type and value to be the same true.
var x = 8;
var y = "8";
x === y; // false
An exclamation point followed by an equal sign (! =) Means that it is not equal in JS. This is the exact opposite of “==”, and only the value will be checked, not the type.
var x = 50;
var y = 50;
x! = y; // false
An exclamation point followed by two equal signs (! ==) means strict not equal. This is the exact opposite (===) and will check both the value and the type. Another familiar character, less than (<), will check if the value on the left is less than the value on the right. Less than or equal to (<=) is the same as above, but equally, it will have the value “true”. More than (>) will check if the value on the left is greater than the value on the right. A value greater than or equal to (> =) is the same as above, but it will also be true.
Dynamic type checking
JavaScript data and variable types are the main programming language. Many runtime errors in JS are type errors. For example, when they try to multiply “a number” by “a string”, they get an error in the form of “Not a Number” of the returned value. Sometimes when calling functions they get the error “undefined is not a function”, this happens when they try to access a property that is not defined. Since JS cannot find the property, it returns its default value to the backup: undefined. Another common type-related error is when trying to change or access a property from a value that is null or undefined. There are no constructors like Undefined or Null.
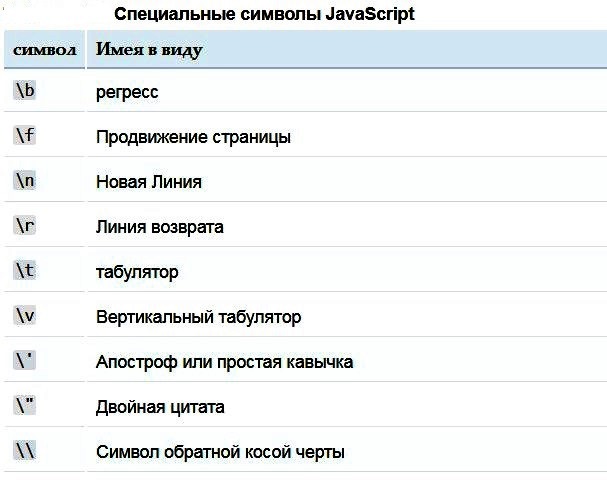
The JS type system helps to avoid these common errors. By definition, it is an interpreted and dynamic language, and requires the type system to work during code execution. The language also tries to help by silently converting value types. Type change or coercion is the reason many developers switch to strict use (===) to check for equality of values.
Dynamic JavaScript data type checking is done through the TypeRight library. Among other features, it uses an approach to implement the following pseudo-classes whose sole purpose is to be the right sides of the instanceof operator:
- PrimitiveUndefined.
- PrimitiveNull.
- PrimitiveBoolean.
- PrimitiveNumber.
- PrimitiveString.
- PrimitiveSymbol.
TypeRight does not currently provide a class for checking whether a value is an object, but it can be easily added.
Variable containers
Variables in JS are containers that contain reusable data. Prior to ES2015, JavaScript variables were declared exclusively using the var keyword:
Today, variables are set by types: var, let, and const. They are unique in their own way and serve to increase the efficiency of code development, however it is recommended to use: let - whenever possible and const - whenever the value of the variable should remain constant. Using variables to store data is the foundation of the language and consists of three parts.
Variable declaration - here the variable is registered in the corresponding scope. Examples of variable declarations:
Initialization occurs when a variable is declared. Here she is assigned memory or space using the JavaScript engine. Because of this, once a variable is declared, it takes on the value undefined even before assignment.
Assigning a variable is the most important step to use. Here, the variable is assigned data that is values using the assignment operator "=".
Values in JavaScript accept one of the standard data types:
- line;
- number;
- logical;
- zero;
- vague.
The syntax for assigning types can be seen above only with strings having single or double quotes. Boolean values can also be either true or false. When naming variables in JavaScript, certain rules must be followed, namely:
- Names must begin with a lowercase letter.
- Names cannot contain characters or begin with characters.
- Names cannot begin with a number.
- Names can contain a combination of uppercase strings, lowercase strings, and numbers.
JS initialization methods
A variable is initialized when its contents or value is set for the first time. For example, price = 22.55 - this may be a way to initialize a variable. It can be declared and initialized at the same time. For example, you can write the variable "price = 22.55" with which the variable was declared and initialized in one line. JS does not require a variable type declaration and even allows a variable to store contents of different types at different times. For example, you could use "price = 22.55", and in a later place write price = "very expensive." This is something that in other languages may lead to an error, but JavaScript is accepted.
JS allows you to use a variable without declaring it. In many programming languages, it is necessary to declare a variable before it can be used, but JS does not bind. When the language finds an undeclared variable, it automatically creates the variable and allows its use.
Incorrect use of the “var” keyword can lead to an error that causes JS to stop working, that is, the web page will not display correctly, var is used only to declare a variable, it cannot be used for anything else. Once a variable has been declared, it will be used without the var keyword preceding it. If the variable is already declared, JavaScript will try to continue and get it.
Work demonstration
You can demonstrate the work of the code in order to consolidate the basic concepts of JavaScript data types; the program reads the code shown in the figure and saved in the html extension file.
After visualizing the result, make sure that the web page displays normally, that is, JavaScript runs fine, and the expected result should show the following:
- Variable price is valid: undefined (accept).
- The variable data1 is valid: null (accept).
- Variable price valid: 32.55 (accept).
- Double price: 65.1 (accept).
- The amount received as a result of multiplying the price by the amount is: 325.5 (accept).
- Variable price now: very expensive (accept).
- Double price now: NaN (accept).
- The price variable was announced for the second time and is now valid: 99.55 (accept).
- Variable $ discount_applied: 0.55 (accept).
Understanding how the language works will automatically make the developer a better programmer. But given that “practice improves,” it’s not enough to know only the theory; it’s important to start putting into practice the basics of the JavaScript database and the functioning of the data. After reviewing the above information, it is not difficult for a novice programmer to answer the question of how many types of data are in the JavaScript language.