Arrays in the Java and C # programming languages are an ordered data set. It consists of elements accessed by indexes. An array in the Java and C # programming languages can be represented as a tape of the same-sized cells. It has a type defined by data. For example, there could be an array of numbers, strings, or objects. Therefore, all cells are the same in size, since the same data type is stored in each of them. If you need to store different data, then you need to make several different arrays.
Ease of using arrays in the Java and C # programming languages
The fact that the cells are the same size allows you to conveniently move around them. The programmer has indexes for access, but since it is known that all data is stored together and physically located in a certain sequence, it is easy to calculate where the necessary data is located. Knowing about this and how much memory each cell occupies, it is easy to find out the location of certain information of a given type. For example, a number is stored in a cell, and we know that 1 cell takes 8 bits, that is, one byte. Then, in order to go to cell No. 3, from the very beginning of the array we need to count two bytes of information, or 16 bits. All these rules and limitations when working with arrays in the Java and C # programming languages are very convenient and help to easily navigate the data.
Arrays in Javascript and their features
With Javascript, things are different and the definition of an array has nothing to do with the one above. The array here is an object. It resembles a list of elements and has additional properties and methods. The type and size of elements in arrays in Javascript are not fixed, and the “length” of the array itself does not become its upper bound. This means that different types of data can be stored in one array: numbers, strings, objects, etc. Nobody can control in terms of language what will be stored in one block. Cell size is also not fixed due to this property of the Javascript array. There is a concept of “length”, but it’s just some kind of property of an object that you can work with in a certain way. There is also the concept of an associative array in Javascript. Such objects consist of key-value pairs. The values in them are associated with keys. That is, when accessing them, the key value is returned. An associative array in Javascript is one with strings as keys.
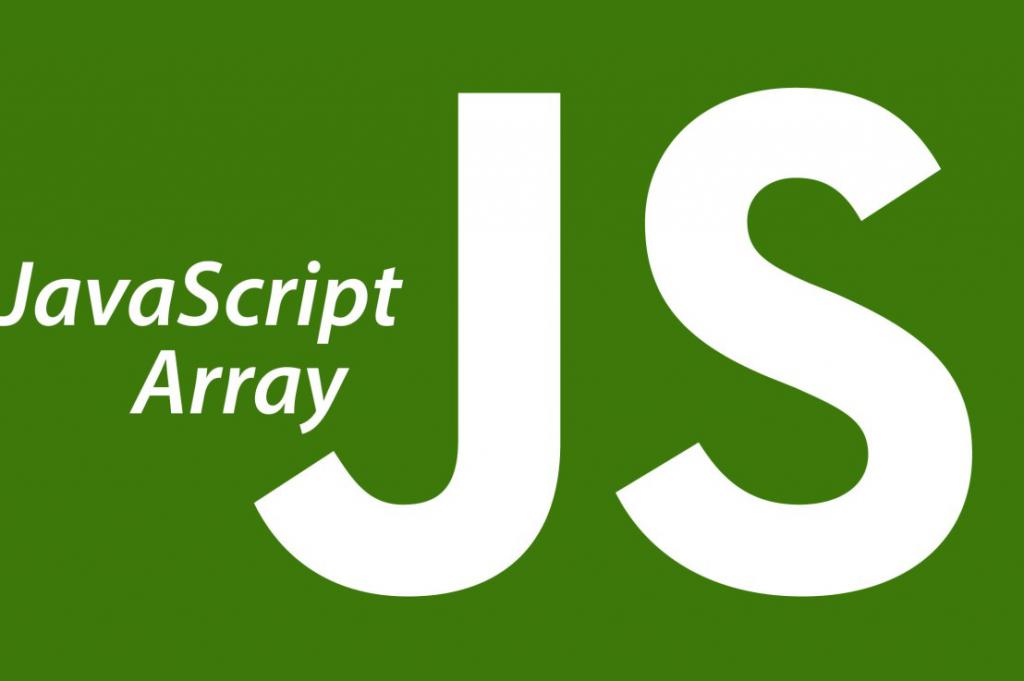
How to create an array in Javascript
To create an array, you must first declare the variable myArray as follows: var myArray = []. We will get an array without elements. Using the classic square brackets that are used in most programming languages, an empty array is created. Now you can work with him. Another option is to create an array with data and immediately populate it. This may be a listing of cities, months, and other values. All of them will be located in the Javascript array in a specific order. The first element has the index “0”, since in programming the numbering always starts from zero, the index of the next element is “1”, and so on. This is the recommended way to create an array using square brackets.
Option to create an array using the constructor
Another option is to create an array using the constructor with the Array function. In this case, a variable is declared, for example, “a”, and using the keyword “new” the value “Array” is placed in it. To name variables in simple letters is not considered good practice, so assigning such values is best only for educational purposes, and not in working with code. And one more note: you need to be careful and careful with the constructor, since with it you can transfer not only a list of some values (for example, strings) - sometimes only one number is present in such a constructor. But this does not create an array with data corresponding to this number. If you write a line of code: “var a new = (5)”, then 5 empty cells simply appear in the array. When outputting to the console, we get square brackets with empty spaces, separated by commas. If you write “1,3” through the comma, you get an array with two elements, the first of which is equal to one, and the second to three. When accessing this array, it is displayed in the console as a list of elements in the following form: [1, 3].
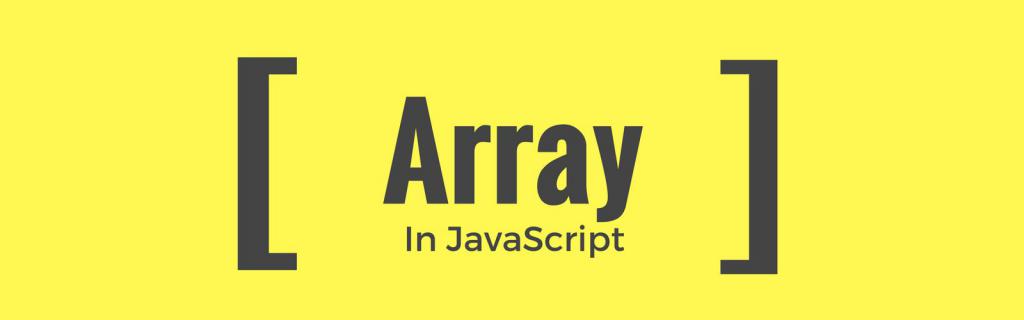
Array length
Arrays in Javascript have a Length property. It means the size of the array, its length. But this method does not always work correctly. In some cases, the actual length of the array will be completely different. Therefore, the programmer needs to understand and remember that this property does not really speak about the real number of elements, although in most cases this is exactly so. Length is the “last element index + 1”. Therefore, if we compare this property with a similar one in C #, then this would correspond to reality. And “index of the last element + 1” would always mean the number of elements in this array. But working with arrays in Javascript is different from other programming languages.
Change array length
The value of the length of the array can be changed manually. For example, you can create a simple array with three identical elements and print the length of its string to the console. She will be equal to three. If we now turn to the various elements of this array, we will get their values. But if then we turn to an element that has no value, we get “undefined”, which means “not defined”. And in this case, the unusual behavior of the property of the length of the array begins.
How the number of elements in an array is calculated
Despite the fact that after the element with a certain index, for example, the third one, there is no other data, you can set the value of the element with the index “10”. This value is assigned to an element with absolutely any index. Then we get a lot of undetectable values until we get to the element with the given value. Thus, although in fact we can have only four elements in the array with many undefined between them, the string length will be equal to “11”, because the index of the last element turned out to be “10”, and the value of the property is calculated as “index of the last element + 1 ". Therefore, if you need to find out how many elements with data in the array, in fact, excluding undefined ones, you do not need to rely on Length.
Features of triggering the Length property
If, as an example, we again turn to the array that we specified with new and try to print its length to the console, then we get 0, since it did not contain elements. But if you apply the same property to an array created using the constructor, then, despite the fact that it is empty, the length of the string will be equal to the number that was in parentheses. Length will still count all elements, even if they are empty.
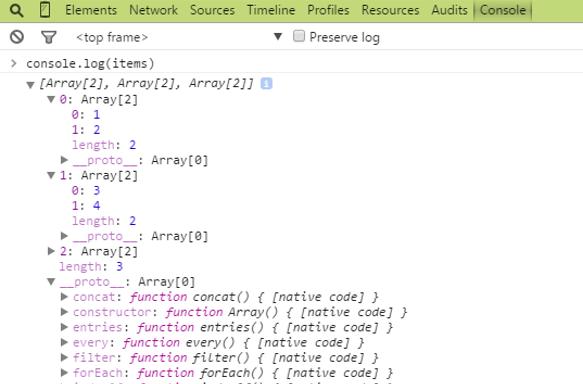
For an array that contained a list of several non-empty elements, this value will be equal to their number. The Length value can be changed by adding the desired value after the “=” sign. And if we change it to any value less than the current, then all the elements that will follow it are cut off from the array. For example, if the length of the array is five, and the Length is two, then all elements after the second will disappear when accessing it. This is one of the easiest ways to cut off the part of the array located at its end. Search in the Javascript array is done using the find function and the indexOf method of the Array object.
Multidimensional arrays and their creation
It's easy enough to create a multidimensional array in Javascript from objects and other elements. Since the values of the elements are not fixed in any way, you can put a number, an object, a string and another array into one array, at the same time. As a result, we get an array with four values, each of which will be a separate type. This way you can create multidimensional arrays in Javascript.
If there are several in one array, then the first will correspond to a zero index, the second to the first and so on. That is, each of them will be displayed as an element. A two-dimensional array in Javascript is a multi-dimensional array with 2 levels of declaration. There are also three-dimensional, four-dimensional and so on. In order to get some part of the array inside another array, its element must also be accessed by index, for example: a [0] [1]. A programmer can create multidimensional arrays in Javascript with an almost infinite number of elements.
Adding and removing an array element
Since an array is an object, the values of its elements are properties of objects. Therefore, you can work with them in a similar way. An element is deleted from the array using the delete property and accessing the index. In multidimensional arrays, when some part of them is removed, a void forms, which will be separated by a comma. But if during removal you want the other values to move up and there is no space between them, then the Splice method is used to delete the element. It takes two parameters. The first is the index from which to start, and the second is the number of elements to be deleted. This method allows you to delete elements in the array, shifting all subsequent elements in such a way that there is no empty space between them.
You can add an element to the Javascript array by accessing the index. The new value is assigned to an existing element as follows: a [0] = 5, where a is the element itself, its index is in square brackets, and after the “=” sign is a new value. A completely new element is also added using an index that is not yet involved in the array. If the size of the array in Javascript is unknown, you can use the Length variable and write it like this: a [a.length] - this will be the number corresponding to the “last index +1”.
Enumerating and sorting arrays in JavaScript
To iterate over an array, you can use a special form of the for ... in loop. But experienced programmers do not recommend using it. The fact is that if you specify an array using the constructor, this method will produce only one digit. When using a counter with a variable from zero to the length of the string, as a result, you can get all the elements at once. Arrays are sorted using the sort method. But in this case, the original value must be preserved if it is still needed in the future.