Java String or strings in Java are the main carriers of textual information, therefore this class is popular and often used. There are several classes for working with strings in the Java.lang package - these are StringBuffer and StringBuilder. They are declared as final - this suggests that it is impossible to inherit from these classes. In order to verify this, open the editor and write the word string, and then go to the class itself. Here we will see that it is final - this means the absence of inheritance.
Ways to create objects
An object of the Java String class can be created in several ways. One of them is using the new keyword and the class constructor. If you press CTRL + P, you can see all of these constructors. Java String accepts a string, an array of char, int, byte, as well as objects of the StringBuffer and StringBuilder classes. The second way to create an object is to directly assign the link enclosed in double quotes.
Classes StringBuilder, StringBuffer and their methods
The StringBuilder, StringBuffer classes are very similar to each other and are close to the String class in purpose. Using methods and constructors, objects of these classes can be transformed into each other and into a string. StringBuilder also has constructors that accept a string and a sequence of characters, so the string can be converted to objects. In both cases, there is a ToString method. It does not apply to the StringBuilder and StringBuffer classes, but to the Object class, with the help of which they can be cast to a string. You can see all the methods if you press CTRL + P. The main difference between the two classes from each other is that StringBuilder is faster, but it is not thread safe, unlike StringBuffer. Thread safety is the ability of code to function in multiple threads at once without blocking.
Features of creating an object with StringBuilder or StringBuffer classes
When creating a StringBuilder or StringBuffer object, the default constructor automatically reserves some memory for 16 characters. This can be checked using one of them, the Capacity method and output to the console. As a result, the buffer size will be displayed - 16 characters. You can press CTRL + P again, and select the Int Capacity method. That is, in the constructor we can set some buffer size. There is also a way to ask it later. To do this, select the Ensure Capacity method in StringBuilder. Using it, we can set the minimum guaranteed buffer size. If you change it, for example, to 20, and then start the console again, we get 34. The thing is that you can specify the minimum guaranteed size, that is, in any case, it will not be less than 20. But, if this A class has a formula in some method or constructor that calculates the allowable value.
Assigning a String Value to an Object
In order to assign a string value to a specific object, you must write this value to the constructor or use one of the Append methods. There are a lot of them, some of these methods can take a boolean, and then convert it to a string and add, some take a char, array, sequences, etc. Unlike String, StringBuilder and StringBuffer, when their methods are used, we continue to work with the same object, rather than creating a new one.
Differences between String and StringBuilder Methods
When working with a string, if you use some methods, for example, concatenating or gluing objects, then in this case a new line is created as a result. You can verify this when printing to the console. Typically, the StringBuilder and StringBuffer classes are used when string addition is very common. For example, in a cycle where there is a large amount of information and the addition of lines constantly occurs, it is better to use them. This will speed up the process, since StringBuilder is doing everything faster, there is no constant creation of a new object.
SetLength Method
When adding lines (with each addition) a new object will be created. If there are more than a thousand, then a lot of extra resources will be spent, and the process itself will take a lot of time. StringBuilder also has a SetLength method, where you can set the buffer size. For example, if you set 15 and print the result to the console, we get the number 20. This object, upon reaching the required buffer size, automatically increases according to the formula specified in this method. The increase can occur almost to a certain limit. For example, in the Capacity method, the maximum number of characters in an object will be equal to the number int.
Insert and Delete Methods
The next method most commonly used with StringBuilder is Insert. It allows you to insert a character at a specified position. He also has many options. Another popular method is to remove a character from a string. To perform this operation, you need to turn to Delete and select some option from the list. For example, you can refer to a substring from the start index to the end, or delete a character using its index.
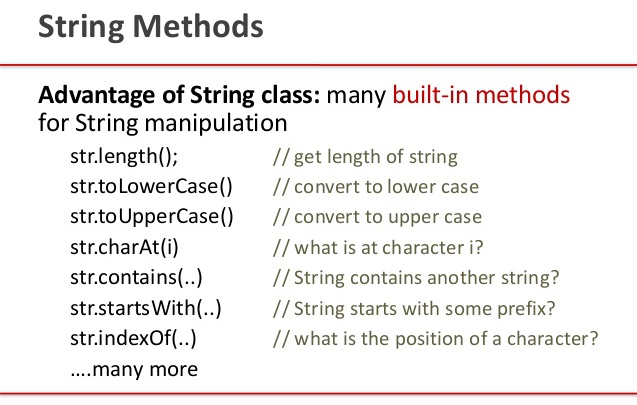
The last StringBuilder method we will parse is Recerse or reverse. It is used to flip a line. The characters in it will line up in reverse order. If you execute it and display the object in the console, we will get its mirror image. In this case, we continue to work with the same object, while a new one is not created. It just simply stores other information. StringBuilder has a lot of methods that are similar to what String has. They work in exactly the same way. There are practically no differences between StringBuffer and StringBuilder by methods, except for StringBuffer thread safety. Due to this feature, it works a little slower.
String class methods
Let's talk about the methods of the Java String class. First, consider them for the srt1 and str2 objects, and then the static methods of the String class itself. The first method that we will parse is CharAt. It takes an index and returns a character. In Java, strings are indexed, that is, there is a symbol under each index. For example, if you create a Java object, then “j” will lie under the index “0”, “a” under the index “1”, “v” - “2”, and “a” - “3”. That is, if you write “0” in the method, it will return the character under the zero index in this line. You can check the operation of this method by outputting to the console and launching the application. In the first case, we will see the symbol “j”. If you change “0” to “1”, then we will see the symbol “a”.
CodepointAt Method
The next Java String method we'll meet is CodepointAt. It returns an int and accepts an int index. CodepointAt returns a Unicode character at the specified index. That is, if we write the index “1”, again referring to the row index and the character “a”, this method will return us a Unicode number corresponding to the character “a”. If we start the application, we will see the number 97. For example, if we know that the index “A” corresponds to the number 65 in Unicode. Then, at startup, where such a value is written in the method, we get 65.
String Comparison in Java String
Another method is compareTo. It returns an int, and takes another string. In Java String, string comparisons are performed using this method. He takes str1 and compares it with the received string, lexically or lexicographically, case-sensitive, that is, taking into account uppercase and lowercase letters. If the contents of the lines are the same, then we should get “0” when starting the application, since characters are subtracted in the process. Therefore, if the contents are different, a certain number equal to the difference of the indices will come in the answer.
Methods compareToIgnoreCase and Concat
The next method we will parse is compareToIgnoreCase. It is similar to the previous one, only here it is not case-sensitive, that is, it doesn’t matter whether it is a capital letter or a capital letter. In the code editor, write str with the Java value and make the last letter capital. And as a result, we still get 0. We will get a different value if there is some other character. Now consider the method for a Java String - Concat. It is designed to add, merge, or combine two lines. Concat is similar to the usual addition of lines using the “+” sign.
Equals and EqualsIgnoreCase Methods
The Equals method accepts another object of type Object. But since all of our inherited classes are of type Object, it means that we can also pass a string. The return type of the Equals method is boolean, that is, our string is compared with another object for equality. The contents of two objects are compared. That is, if we pass the string str2, then if its contents are not equal to the object, we will get the answer false, and if it is equal, then true. There is also a case insensitive string comparison method. It is called - EqualsIgnoreCase. It is similar to compareTo and works in a similar way. The return type here is boolean. In the case of compareTo, we end up with a character difference of either 0 if the content is the same.
HashCode and IndexOf Methods
The next method is HashCode. It returns the hash code of our object. Each object has it, and represents a number, more precisely, a bit string of a fixed length. You can get it using this method. Consider the IndexOf method. Actually there are several of them, but we will consider only one. It takes a variable ch - a Unicode variable. That is, if this variable matches the character in the string, then the index of this character is returned, if not, then “-1”. If our Unicode number is on this line, then the index of this character is returned. If there is no such character, then “-1” is returned.
Methods IsEmpty, Length, Split, Substring
Now let's parse the IsEmpty method. It returns true if the length of the string is 0, i.e. it is empty. The next method is Length. It is responsible for the number of characters in a string, that is, it returns the length of the Java String. Spaces are also characters. The Split method takes a separator string and returns an array of strings. We can use a literal placed in double quotes, or a string. When a method encounters a space, it divides the string and distributes the parts into an array. As a result, we get an array of strings. We can put a comma in the string, Split will also split it into parts. We turn to the Substring method. It extracts a substring from a string, endindex-beginindex long, starting at the last position. One example of a Java String for this method is to write a string and set two indexes for it, then the string will be cut and its beginning will be the first index and the end the second. The console will display a specific part of str1. To wrap a string in Java String, "\ n" is added.
Methods ToCharArray, ToLowerCase, ToUpperCase, Trim
The ToCharArray method returns an array of characters, that is, the string is parsed character by character. If you declare some kind of char, and then put a certain element in the method, then each character will be in the char array. Then each element of the array is displayed in the console. Another method is ToLowerCase. It translates the string to lowercase. ToUpperCase works in the opposite way - it makes all the letters in the string uppercase. A very commonly used method is Trim. It truncates spaces at the beginning and end of a line. There are a lot of static methods of the class itself, because they accept different types and arrays. The most commonly used is ValueOf. It converts a variable of the given type to a string.