The Python programming language is a powerful tool, famous for its beautiful syntax and ease of learning. Literally any user can learn the basics of this language in a short time and write his first program. In this article, we will look at sample beginner programs in Python.
Why Python?
Many have difficulty choosing the first programming language. Here are a few main reasons why you should opt for it:
- Easy to learn. Indeed, Python is a very light language. You can learn the basics in just a week. Some are of the opinion that if a person chooses Python as his first language to learn, then it will be difficult for him to learn other languages in the future. But if a person does not understand programming at all, does not know how everything works, it will be very difficult for him to learn Java, for example. First, the user must understand the basics of programming, find out what OOP is, how to work with it.
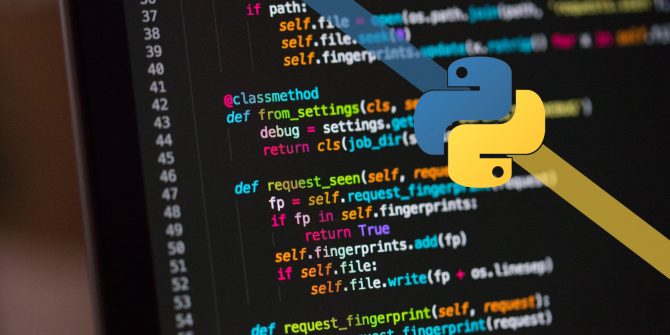
- Perspective. Today, many IT companies are switching to Python. Sites are written, calculations are made, bots are created. "Python" copes with all the tasks. And a good specialist in this area will not be lost. There was little talk about this language before, but now even in schools they are replacing Pascal with Python. When passing the exam, you can solve problems on the "Python".
- A lot of training material. Different courses, books, lessons, sample programs. You can even find sample Python programs for the Raspberry Pi. This is such a microcomputer, which is often used to build smart homes, automatic systems.
Which version of Python to choose
There are two versions of Python - 2 and 3. Beginners when downloading Python are wondering which version is worth downloading. The second version is already outdated, and few people use it. Most lessons and sample Python programs are version 3. And this article also uses Python 3 in the examples. The syntax is not very different, but some libraries do not support the old version, some have a slightly different syntax. Therefore, you should not waste time on the second version, you need to immediately download and study the third.
Very first program
This tradition has developed among programmers that the first program in any programming language is the output of the text Hello World !, which translates from English as “Hello World!”. To accomplish this task, you need to register only one statement - print. And in parentheses write in quotation marks the text that you want to display on the screen. So our first example Python program looks like this:
print('Hello World!')
Any program works according to a certain algorithm. The main ones are: linear, branching, cyclic. The very first example of a Python program that was considered, that is, text output, refers to a linear algorithm. Often, programs use all kinds of algorithms. Below are examples of simple Python programs related to other algorithms.
Branching
A branch or branching algorithm must contain at least one condition check. To check conditions, Python has an if statement, which translates as if.
a=0 print(' : ') input(a) if a==0: print(' 0')
In the example above, you can see an example of working with the IF statement in Python. First, the program asks for a number, then the user enters a number (input operator). This number is written to the variable a. The following is the condition, if the variable "a" is zero, then the variable displays the text on the screen, after which the program stops. There is also an else statement that translates to “different”. We supplement our program in such a way that if a person enters a number other than zero, she will notify the user about this by displaying the text. So, the finished program is as follows:
a=0 print(' : ') input(a) if a==0: print(' 0') else: print(' ')
Python loops
Cycles serve for repeated repetition of any action. In Python, there are for and while statements for this. Consider both of these operators.
While statement
These are cycles with a condition, that is, the body of the cycle will be executed until the condition is true. For example, while a = 0, add the variable c to the variable b.
The syntax is as follows:
while :
An example Python program using a While loop:
a = 1 while a <= 10: print(a ** 2) a += 1
This program displays squares of numbers from 1 to 10. In the body of the loop, you can see that the program displays the variable a squared each time, then adds 1. The cycle is executed until the variable a is equal to or greater than 10. To make the program look more complete and beautiful, you can use the else operator. Usage example:
a = 1 while a <= 10: print(a ** 2) a += 1 else: print(' ')
That is, if the variable a becomes greater than or equal to 10, then the message "Cycle completed" is displayed. We added only 2 lines of code, and the program looks more beautiful. And besides, in the future it will be easier to navigate, if there is any mistake, you won’t have to look long.
Operator for
The For loop is less versatile than the While loop, but it works faster. Basically, using for iterate over any data. For example, strings and dictionaries. Example Python program:
for i in 'Hello world!': print(i * 2, end='')
In this example, you can see the for loop iterate over the string Hello World! and each character of the string repeats twice.
Break and continue statements
The break and continue statements are used in loops to exit a loop or skip to the next iteration. An example of using the continue statement:
a = 1 while a <= 10: if a==5: a += 1 continue print(a ** 2) a += 1 else: print(' ')
In the example, we see that if the variable a is 5, then it skips 5 and starts with 6. The break statement is also used, but instead of skipping, it will exit the loop and move on to other actions, if any. If they are not, terminates the program.
Graphical program interface
The following are sample GUI programs in Python. To create them, you need a set of PyQT5 libraries. This set is one of the most powerful for creating a graphical interface of the program. There is also a TKinter library for creating a graphical interface, but it is inferior in power to PyQT5, although TKinter can also be used for simple programs. To begin with, you should install this library, since initially it is not in Python.
The simplest example Python program with PyQT5:
Everything is very flexible, you can adjust the screen width, height, window title and much more. Below you can see the window that is created after executing the code above.
Math library example
The Python programming language is often used for calculations. Even NASA uses Python for such purposes. To carry out operations with numbers, carry out various calculations, the math library will help. This is a very powerful library, which initially comes with Python, and you do not need to install it additionally. Let's look at a couple of examples of using this library.
Suppose you need to calculate the factorial of a given number. This can be done only by the operator. Example Python program:
import math a=10 print(math.factorial(a))
Find out the remainder of dividing a by b:
import math a=10 b=2 print(math.fmod(a,b))
Suppose we need to calculate the inverse hyperbolic cosine of a number B, this is also done with a single operator:
import math a=10 print(math.acosh(a))
Plotting
Python can also make graphics. For this, the MatPlotLib library is used. This library does not exist initially, it must be installed separately. This is done very simply, on the command line you need to write one line:
pip install matplotlib
Then you should wait a while until the library is installed.
Sample code for plotting sine and cosine graphs:
import matplotlib as mpl import matplotlib.pyplot as plt import math dpi = 80 fig = plt.figure(dpi = dpi, figsize = (512 / dpi, 384 / dpi) ) mpl.rcParams.update({'font.size': 10}) plt.axis([0, 10, -1.5, 1.5]) plt.title('Sine & Cosine') plt.xlabel('x') plt.ylabel('F(x)') xs = [] sin_vals = [] cos_vals = [] x = 0.0 while x < 10.0: sin_vals += [ math.sin(x) ] cos_vals += [ math.cos(x) ] xs += [x] x += 0.1 plt.plot(xs, sin_vals, color = 'blue', linestyle = 'solid', label = 'sin(x)') plt.plot(xs, cos_vals, color = 'red', linestyle = 'dashed', label = 'cos(x)') plt.legend(loc = 'upper right') fig.savefig('trigan.png') plt.show()
After executing the code, you can see the following:
Django
There are many websites written in Python. More and more sites are written every day in this wonderful language. And the possibility of this is provided by the Django framework. They even suggest that in a few years Python will be able to overtake PHP in the market. Many IT companies already work with this language. For example, Yandex. And the well-known cloud service Dropbox is completely written in Python. You can write not only sites, but also full-fledged web applications. In addition, it is absolutely free.
Python programming IDE
A programmer’s tool is always important. Agree, it is inconvenient to program in the Python programming language in notepad, especially considering how "Python" refers to indentation. There are several development environments that are most commonly used:
- PyCharm. This is probably the most famous IDE for Python. It’s very convenient to work with it; connecting new libraries does not take much time. But it is more suitable for powerful computers, on weak PCs it will be very inconvenient to work with it, since PyCharm is demanding.
- Sublime Text 3. This is not a development environment, it is a code editor, but what a! It is ideal for low-end computers. Program codes will have to be run through the command line.
- Eclipse It is most often written on it by programmers in Java, but for Python it is perfect.
Recommendations
- Do not forget to comment on your code. Many do not spend time on this, but in vain. You need to have a habit of commenting on your code, and always. For example, if you post your example Python program on some Internet resource, other programmers need to understand your code, this is very important.
- Practice and read books. Practice is always needed. Participate in Open Source projects, solve problems. Also, don't forget to look at programming examples in Python by other programmers. You need to learn how to work with someone else's code. Well, of course, you need to read books. Videos, articles are certainly cool, but nothing can replace books.
- Learn to use search engines. Often on the forums you can see that people ask completely stupid things, the answers to which can be found on the front pages of search engines. Almost 95% of your questions can be answered online.
- Do not abuse sample programs. Learn to write code yourself. If you only look at examples of compiling programs in Python by other developers and work with them, you will not learn how to write your code.