Starting to write a program, a modern developer must first think through the architecture of a future project. From the point of view of an object-oriented approach to software development, this means that the programmer must create code that will consist of logical blocks (classes and objects) and think through their interaction. The code must be supported. This means that in the future this program can be painlessly supplemented or expanded without changing its main components.
In the object-oriented development paradigm, there are three main tools that developers use to write good code:
- Encapsulation.
- Inheritance.
- Polymorphism.
- Inheritance.
Inheritance
This is the process of creating a new class based on an existing one. The object-oriented approach is primarily used to describe the concepts and phenomena of the real world with a programming language. Therefore, in order to understand OOP, it is necessary to learn to describe the task facing the programmer, the language of classes and objects.
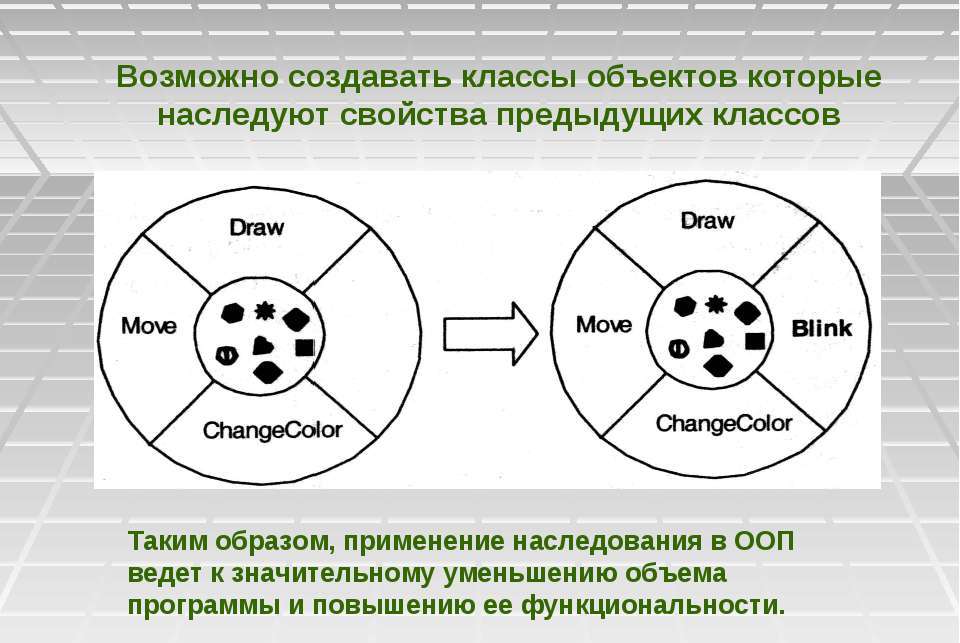
In order to understand the essence of the process of inheritance, we can give an example from life. There is an abstract concept of "man." Any person has common characteristics with which he can be described. These are height, weight, hair color, timbre of voice, and so on. Ivanov Ivan, a student of the 1st “A” class, is a specific representative of the concept of “man,” that is, in the language of an object-oriented approach, the concept of Ivanov Ivan is the heir to the concept of man. Or, for example, the neighbor's cat Barsik is the heir to the concept of “cat”. You can make a more complex inheritance: the concept of Barsik is inherited from the concept of Maine Coon, which in turn is inherited from the concept of "cat".
How is this described by a programming language?
In software development, the inheritance process makes it possible to create a class based on others. The parent class is called the base class, and the remaining heirs are derived. Borrowing is that the methods, properties and fields of the parents pass to descendants. The syntax for class inheritance in the C # programming language might look like this. For instance:
class Ivan : Person { // {
An example of the class inheritance mechanism:
class Person { public int Age { get; set; } } class Ivan : Person { public void Talk() { // } } class Peter : Person { public void Walk() { // } } class Program { static void Main(string[] args) { Ivan ivan = new Ivan(); ivan.Age = 29; // Peter peter = new Peter(); peter.Age = 35 ivan.Talk(); peter.Walk()(); } }
Child classes are derived from the base class Person, and the Age property is available for both classes.
Base class constructor
Creating a class with a declared constructor and a successor class, a logical question arises: what constructors will be responsible for what when creating objects of these classes?
If it is specified only in the inherited class, then when creating the heir object, the default constructor (parent) is called, and then its receiver.
If the constructors are specified in both the parent and the child classes, then the base keyword is used to call the base. When declaring such an inheritance structure, the following syntax is used to specify the constructor:
public Ivan (int age) : base (age) { // }
Inheriting class methods is an essential property of OOP. When creating an object of a successor class, the arguments of the constructor of the base class are passed to it, after which its constructor is called, and then the remaining calls to the constructor of the heir are performed.
Access fields from a child class
Inheriting access to the properties and methods of the base class from the derived class becomes possible if all of them are declared with the following keywords:
1. Public.
Provides unlimited access to class members: from Main, from descendant classes, from other analogues.
2. Protected.
Access to the member remains only from the source class, or from derived analogues.
3. Internal.
A member restriction is localized only by this project assembly.
4. Protected internal.
A member restriction is limited only by this project assembly or from derived classes.
Inheritance of access to class members is dependent on keywords. If a member is not declared as such, then accessibility is set by default (private).
Multiple inheritance
Interfaces is a contract that provides guarantees that a set of methods will be implemented in the class that inherits the interface. The latter do not contain static members, fields, constants, or constructors. It is illogical and you cannot create interface objects.
These structures are created outside the classes, declared using the interface keyword:
interface IMyInterfave { void MyMethod (int someVar); }
The rule of thumb when creating interfaces is to start the name of the structure with a capital letter I, so that they can be easily distinguished in the future. The body of the interface announces the signatures of its members.
To indicate that the class will implement the interface, you must specify the name of the construct after the class declaration in the same way as with usual inheritance:
class MyClass : IMyInterface { public void MyMethod (int someVar) }
Interfaces are needed to implement multiple inheritance. In the C ++ programming language, class inheritance is possible; C # uses interfaces for these purposes. A class can implement a large number of interfaces, in which case they are listed with a comma after the class declaration:
class MyClass : IMyFirstInterface, IMySecondInterface { // }
By default, all properties and methods of interfaces have public access, because they must define some functionality that will be implemented in the class in the future. Inheriting in C # almost always involves using this handy tool.
A little more about class inheritance
Java, C #, C ++ - all object-oriented languages in their own way implement the inheritance mechanism. But the essence is the same everywhere: to transfer the properties of the parent to the descendant object. Interfaces, abstract classes are all convenient tools for implementing this task.